This special app type is used to provide import and export functionality.
IMatch integrates these apps in the Import & Export Panel.
The app.json file
Import and Export Apps are identified by the type
element. The following values are supported:
Type | Description |
---|---|
import | This app is used for import purposes. |
export | This app is used for export purposes. |
| This app is used for both import and export purposes. |
hybrid | This app is used for both import and export purposes and also shows up in the App Manager. When you specify this type, your app needs to be able to handle both execution from the Import & Export panel ( IMatch.getDropContext() succeeds) and from the App Manager (IMatch.getDropContext() fails). |
Any app that with the one types specified above will be listed in the Import & Export Panel in IMatch.
Depending on the value defined in the app.json of your app, IMatch can perform certain internal optimizations while the app is running. Always specify the type that best matches what your app does.
Example
This app.json describes an export app (type
= "export")
{
"type" : "export",
"id": "photools.com.IMatch.HTMLReport",
"version" : "1.0",
"name":
{
"en": "HTML Report",
"de": "HTML-Report"
},
"description":
{
"en": "This app shows how to create a plug-in app for the Import & Export Panel.",
"de": "Diese App demonstriert die Implementierung einer Plug-in App für das Import & Export-Panel"
},
...
Name and Description
IMatch uses the name and description of the app as defined in app.json
as the title and description in the Import & Export Panel.
Drag & Drop
A user can run a module in the Import & Export Panel either by dropping objects onto the module panel, or by clicking the Run button.
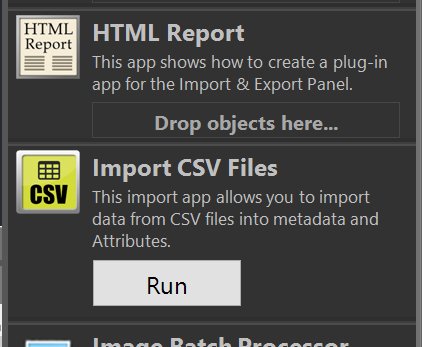
If your import/export app supports dropping of objects, include this in your app.json
file:
"supportsDrop": true
If supportsDrop
is not included or specified as false, the Import & Export Panel displays the app with a Run button (See the CSV Import App in the image above).
Otherwise it shows the Drop objects here... trigger area.
Processing Dropped Objects in an App
The function IMatch.getDropContext returns a Promise and on success a JSON object which describes the objects the user has dropped on your app in the Import & Export Panel in its scope
element.
The general structure of the drop object looks like this:
{
"type": "file",
"recursive": false,
"id": [
638,
1229,
1230,
1231,
1232
],
"textData": [],
"idlistFiles": "@imatch.importExportFiles",
"idlistFilesSize": 5
}
In this example, the user has dropped 5 files onto the module in the Import & Export Panel.
Element | Description |
---|---|
type | The type of the dropped object(s). Supported are: file, folder, category, collection, db and hdrop.db is used when the user dropped the database node. hdrop is used when the user drops files from Windows Explorer. |
recursive | If the user holds down the Alt key while dropping objects, the recursive element is true.Your app is then supposed to process the dropped objects recursively (for folders, categories, collections). |
id | The id(s) of the dropped object(s). If the type is file, it contains one or more file ids. If the type is folder, it contains one or more folder ids... |
textData | If type is hdrop, this array contains the names of the dropped files. |
idlistFiles | This element contains the name of the (temporary) idlist with all dropped files. If the user drops files, this idlist contains all dropped files. If the user drops folders, categories or collections, this idlist contains all files contained in the dropped objects. The idlist responds to the recursive option and e.g., processes dropped folders recursively to get all files.If your app is not interested in the type of the dropped object and just operates on files, you can use this idlist to directly access the files via the /files endpoint.This idlist is only valid for the current import and export operation. |
idlistFilesSize | The number of files contained in idlistFiles |
Usually you retrieve the dropped objects as the first thing in your app. For example:
$(document).ready(function () {
// Get the dropped objects
var drop;
IMatch.getDropContext().then(function(response) {
drop = JSON.parse(response.scope);
if (drop.type == 'files') {
...
}
}, function(error) {
// No scope available!
});
...
Note note that the promise returned by IMatch.getDropContext() will be rejected if you call this function from an app that was not launched from the Import & Export Panel. Or when you run the app in an external webbrowser.
What your app then does with the dropped objects of course depends on your app.
The following code fragment just iterates over all dropped files, logging the file names to the console.
Note how it uses the name of the idlist (drop.idlistFiles
) from the drop object with the IMWS /files
endpoint to retrieve the id and name for each dropped file.
$(document).ready(function () {
// Get the dropped objects
var drop;
IMatch.getDropContext().then(function(response) {
drop = JSON.parse(response.scope);
// Just iterate over all dropped files.
IMWS.get('v1/files',{
// We use the idlist from the drop object
// to tell IMWS which files we are interested in
idlist: drop.idlistFiles,
// We only need the id and the file name
fields: 'id,filename'
}).then(function(response) {
response.files.forEach(function(f) {
console.log(f.fileName);
}, this);
});
});
...
Tip: Simulating Dropped Objects
If you want to test or debug your import/export app in a web browser outside of IMatch, you app does not have access to the dropped objects. You can work around that by simulating a dropped object. Here is an example:
var dropObject = null;
$(document).ready(function () {
if (IMatch.isEmbedded()) {
// Get the dropped objects from IMatch.
dropObject = IMatch.getDropContext();
}
else {
// If we run this in a browser, we simulate a drop object.
// We just use the name of the idlist representing the
// files selected in the file window. Neat ;-)
dropObject = {
type: "files",
idlistFiles: IMatch.idlist.fileWindowSelection
}
}
...
});
Examples
The CSV Import app and the HTML Report app show how to create apps which integrate into the Import & Export Panel.